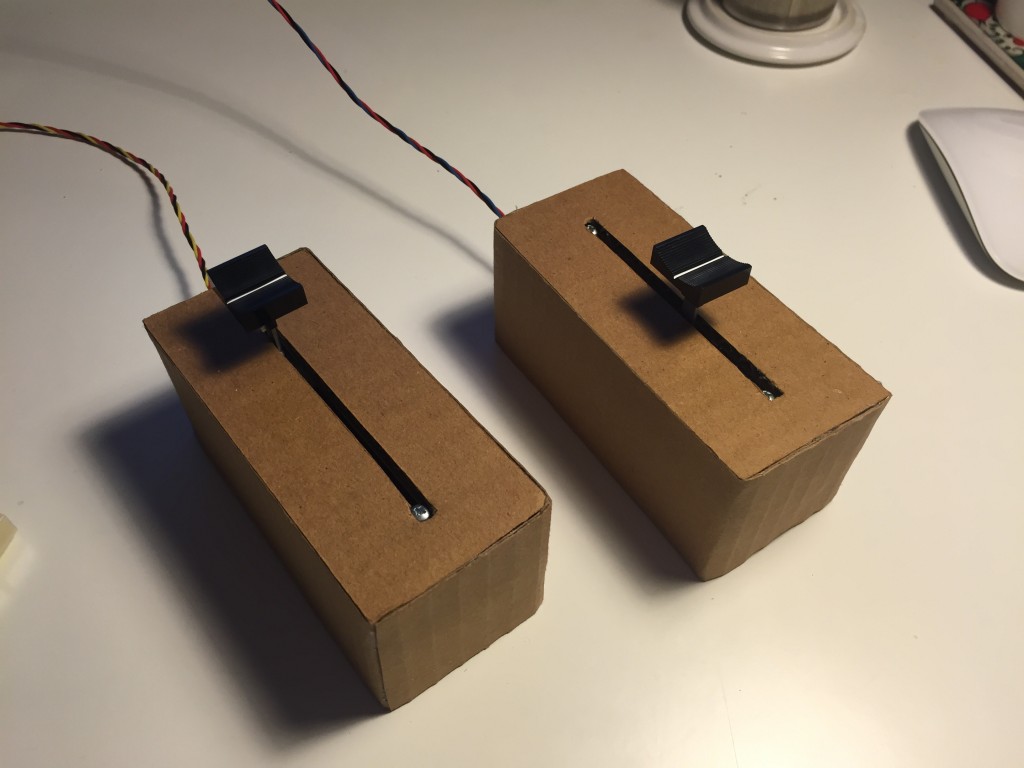
I wanted to make some controllers for the pong game I’ve been plugging away at for ICM. Constructing the prototypes was pretty straight forward, but getting the code to work has been a whole other thing.
I had some slide pots already from a previous project, so all I really needed to do was make a housing for them. Cardboard is free, and I actually quite like it as a material.
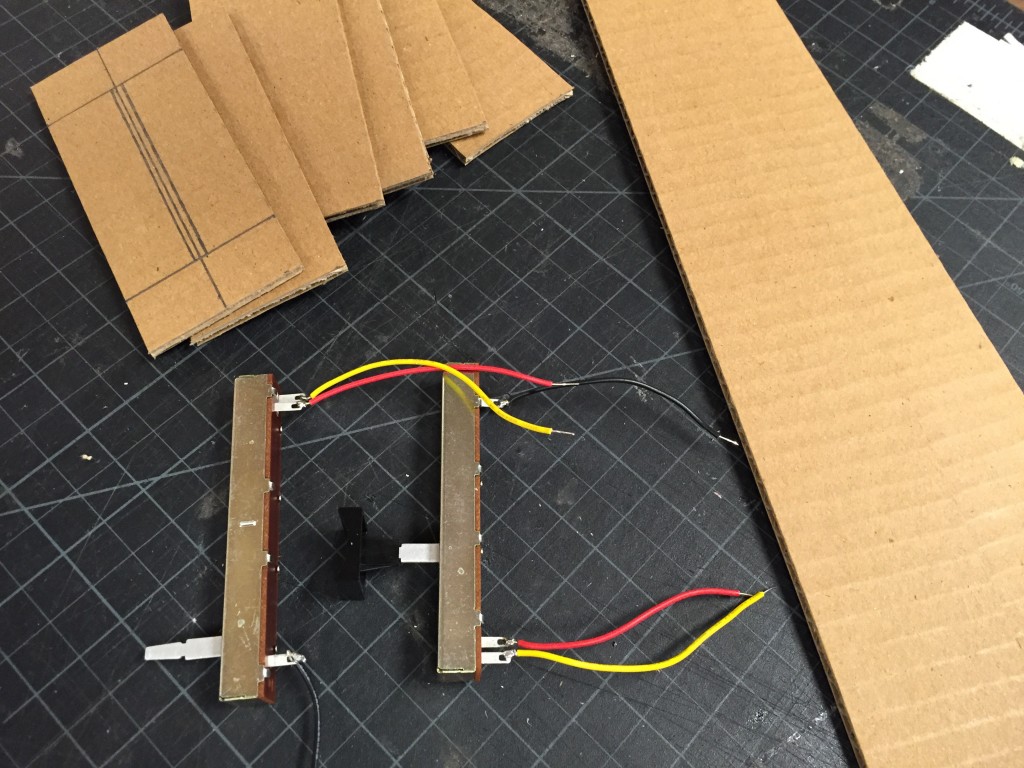
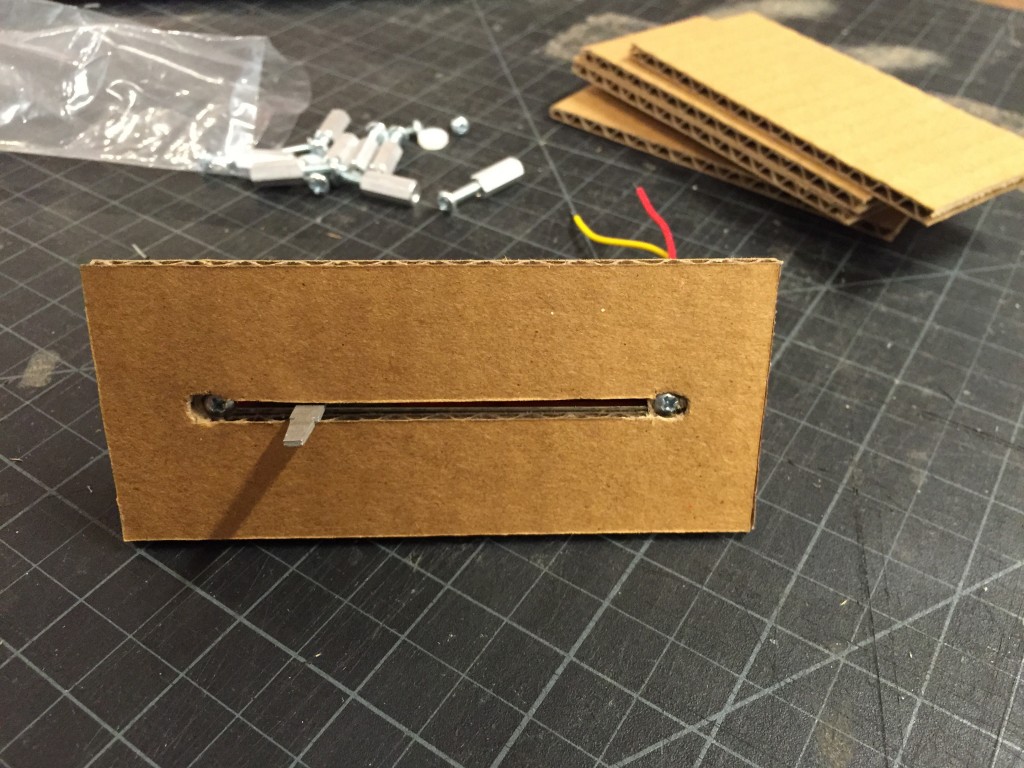
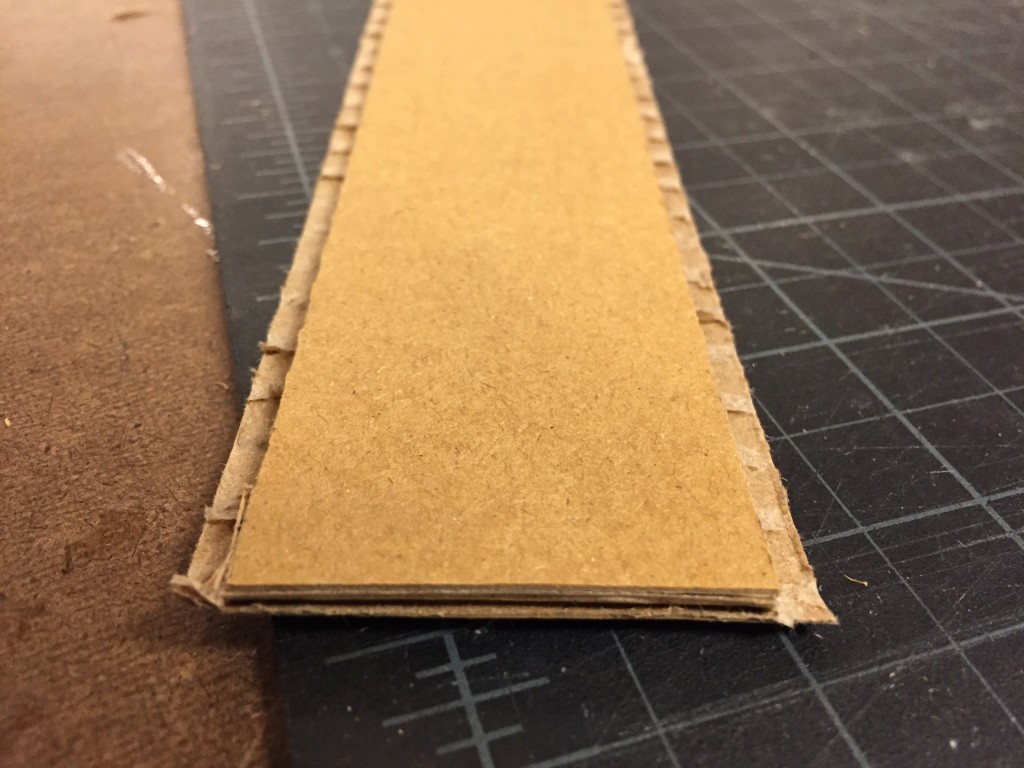
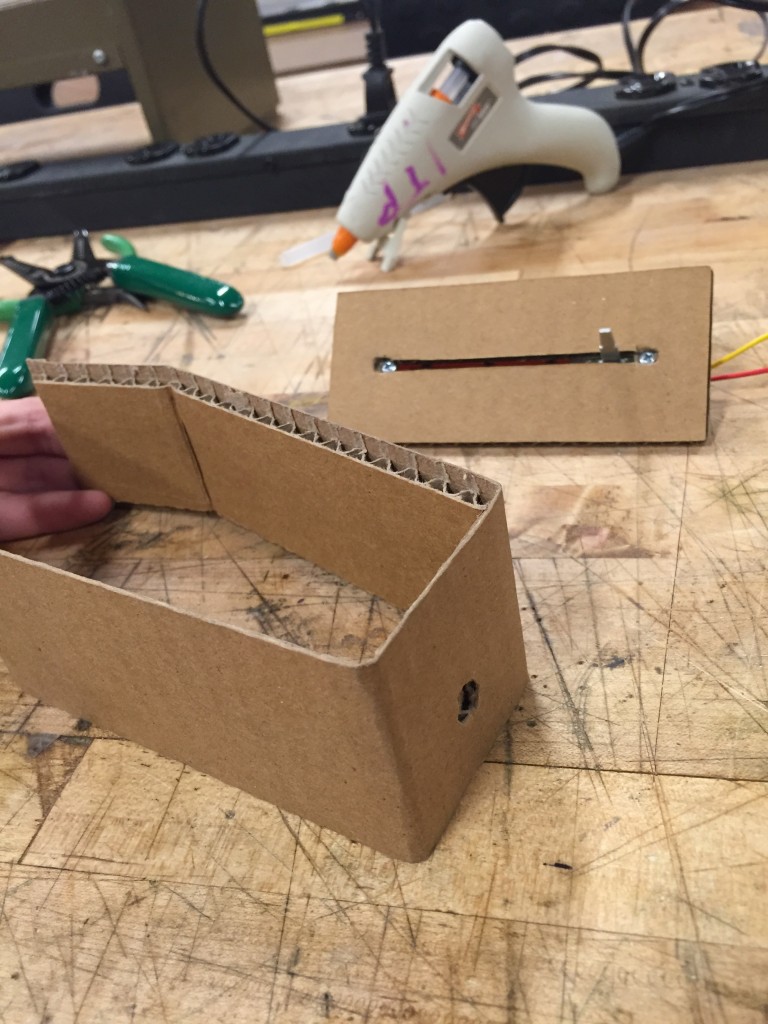
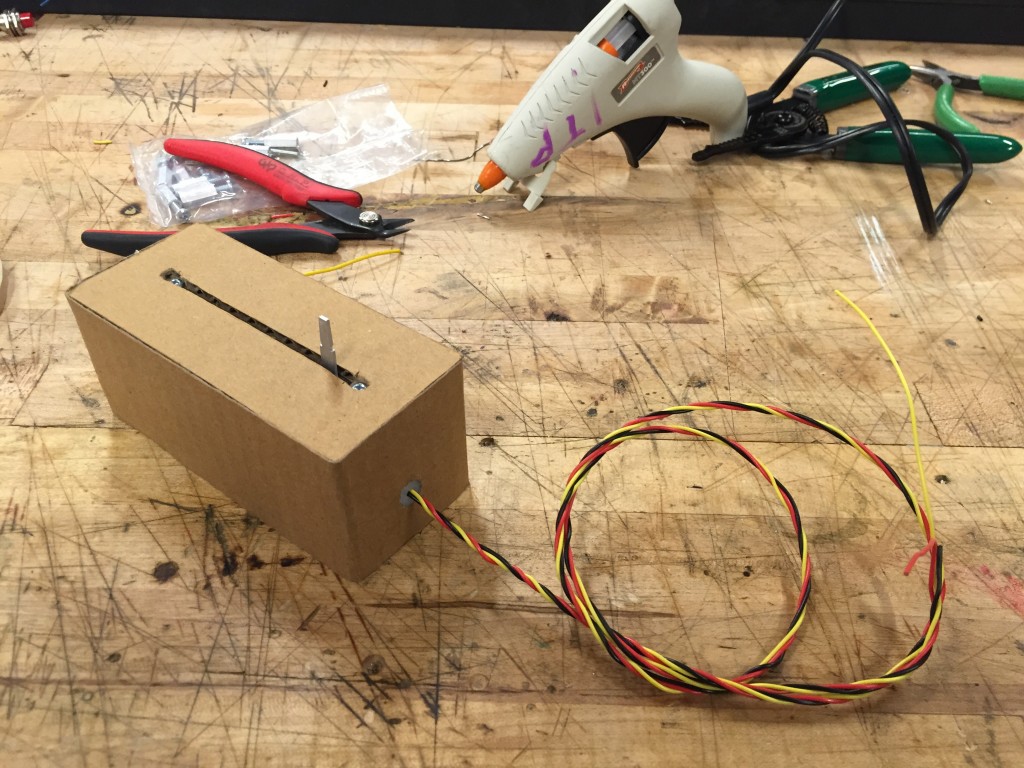
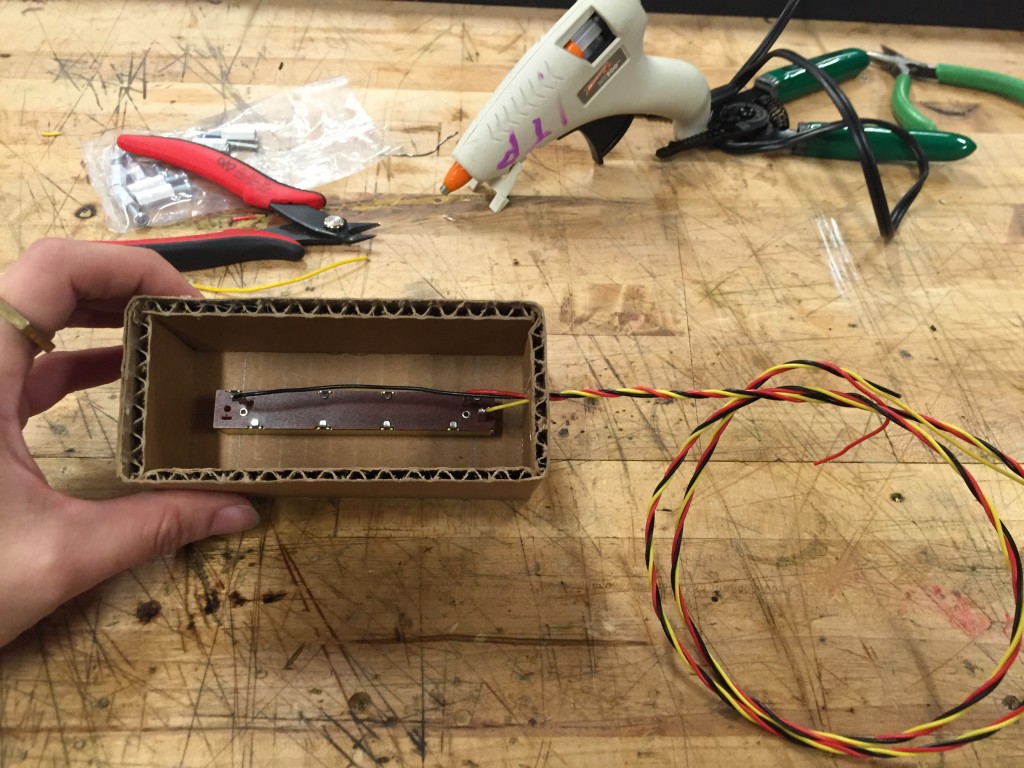
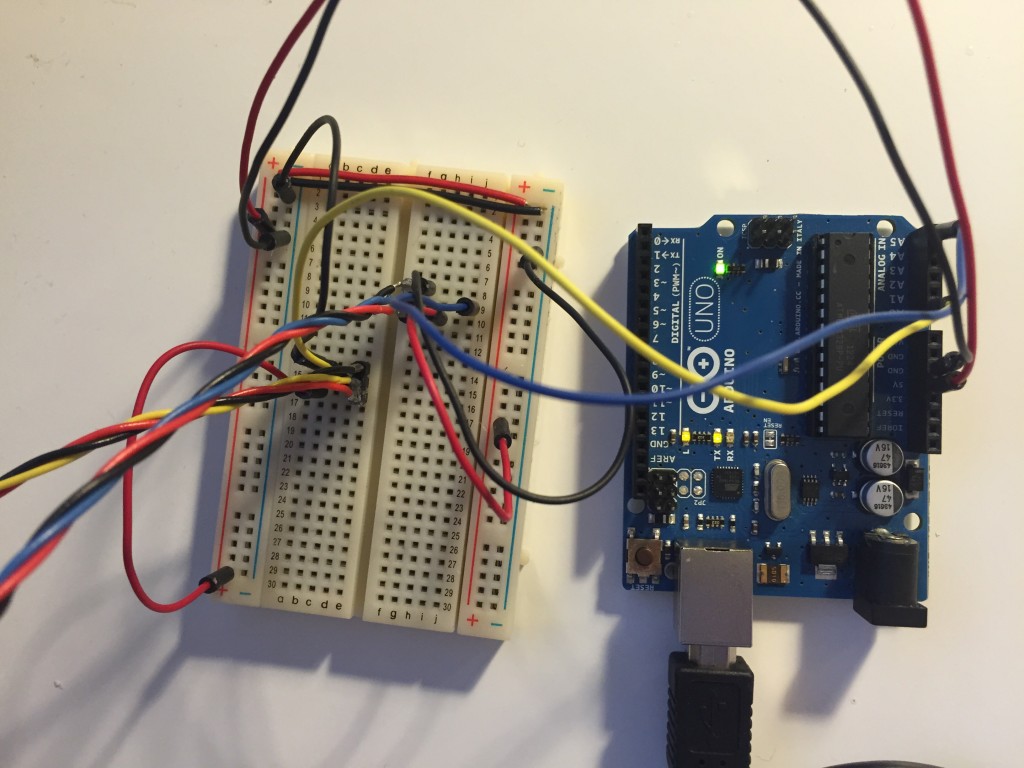
The Arduino code seems to work just fine:
void setup() { Serial.begin(9600); } void loop() { //send two things separated by a commma int slideOne = analogRead(A0); // read the input pin int mappedSlideOne = map(slideOne, 0, 1023, 0, 255); // remap the pot value to fit in 1 byte int slideTwo = analogRead(A5); // read the input pin int mappedSlideTwo = map(slideTwo, 0, 1023, 0, 255); // remap the pot value to fit in 1 byte Serial.print(mappedSlideOne); // print it out the serial port Serial.print(","); Serial.println(mappedSlideTwo); delay(1); }
But the p5.js code keeps coming up ‘undefined’. I can’t figure out what’s wrong with the code. After I’ve gotten that worked out it should be pretty easy to drop it into the pong code I’ve already been working on.
var serial; // variable to hold an instance of the serialport library var portName = '/dev/cu.usbmodem1421'; // fill in your serial port name here var inData; // for incoming serial data var myData; var input; var input2; var inString; function setup() { serial = new p5.SerialPort(); // make a new instance of the serialport library serial.on('list', printList); // set a callback function for the serialport list event serial.on('connected', serverConnected); // callback for connecting to the server serial.on('open', portOpen); // callback for the port opening serial.on('data', serialEvent); // callback for when new data arrives serial.on('error', serialError); // callback for errors serial.on('close', portClose); // callback for the port closing serial.open(portName); // open a serial port serial.list(); // list the serial ports createCanvas(400, 300); } function draw() { background(0); fill(255); //text("sensor value: " + input + "," + input2, 30, 30); text("sensor value: " + input, 30, 30); text("hello world", 30, 50); print(inString); } function serverConnected() { println('connected to server.'); } function portOpen() { println('the serial port opened.'); } function serialEvent() { inString = serial.readStringUntil('\r\n'); if (inString.length > 0) { var parts = inString.split(","); //split the incoming into an array based on the comma input = int(parts[0]); input2 = int(parts[1]); print(input2 + "Got data" + input); } } function serialError(err) { println('Something went wrong with the serial port. ' + err); } function portClose() { println('The serial port closed.'); } // get the list of ports: function printList(portList) { // portList is an array of serial port names for (var i = 0; i < portList.length; i++) { // Display the list the console: println(i + " " + portList[i]); } }